Java 面向对象设计 - Java 终止块
try
块也可以有零个或一个 finally
块。 finally
块总是与 try
块一起使用。
语法
使用 finally
块的语法是
finally {
// Code for finally block
}
finally
块以关键字 finally
开始,后面紧跟一对大括号。
finally
块的代码放在大括号内。
try
,catch
和 finally
块有两种可能的组合: try - catch - finally
或 try - finally
。
try
块可以后跟零个或多个 catch
块。
try
块最多可以有一个 finally
块。
try
块必须有一个 catch
块,一个 finally
块,或者两者兼而有之。
try-catch-finally
块的语法是:
try { // Code for try block } catch(Exception1 e1) { // Code for catch block } finally { // Code for finally block }
try - finally
块的语法是:
try { // Code for try block } finally { // Code for finally block }
无论在相关联的 try
和 /
或 catch
块中发生什么,finally
块都被保证被执行。
通常,我们使用 finally
块来写清理代码。
例如,我们可能获得一些资源,当我们完成它们时,必须释放。
try - finally
块允许你实现这个逻辑。
您的代码结构将如下所示:
try { // Obtain and use some resources here } finally { // Release the resources that were obtained in the try block }
例子
下面的代码演示了 finally
块的使用。
public class Main { public static void main(String[] args) { int x = 10, y = 0, z = 0; try { System.out.println("Before dividing x by y."); z = x / y; System.out.println("After dividing x by y."); } catch (ArithmeticException e) { System.out.println("Inside catch block a."); } finally { System.out.println("Inside finally block a."); } try { System.out.println("Before setting z to 2."); z = 2; System.out.println("After setting z to 2."); } catch (Exception e) { System.out.println("Inside catch block b."); } finally { System.out.println("Inside finally block b."); } try { System.out.println("Inside try block c."); } finally { System.out.println("Inside finally block c."); } try { System.out.println("Before executing System.exit()."); System.exit(0); System.out.println("After executing System.exit()."); } finally { // This finally block will not be executed // because application exits in try block System.out.println("Inside finally block d."); } } }
上面的代码生成以下结果。
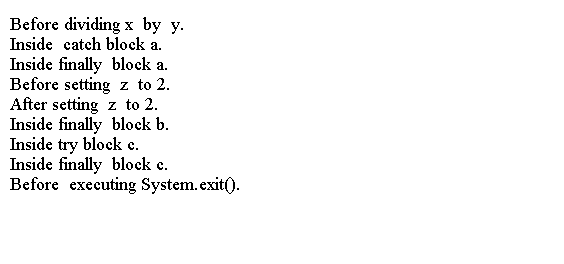
重新引用异常
捕获的异常可以重新引用。
public class Main { public static void main(String[] args) { try { m1(); } catch (MyException e) { // Print the stack trace e.printStackTrace(); } } public static void m1() throws MyException { try { m2(); } catch (MyException e) { e.fillInStackTrace(); throw e; } } public static void m2() throws MyException { throw new MyException("An error has occurred."); } } class MyException extends Exception { public MyException() { super(); } public MyException(String message) { super(message); } public MyException(String message, Throwable cause) { super(message, cause); } public MyException(Throwable cause) { super(cause); } }
上面的代码生成以下结果。
