Java面向对象的设计 - Java异常使用
访问线程的堆栈
以下代码显示了如何获取线程的堆栈帧。
Throwable对象在创建线程的点处捕获线程的堆栈。
public class Main { public static void main(String[] args) { m1(); } public static void m1() { m2(); } public static void m2() { m3(); } public static void m3() { Throwable t = new Throwable(); StackTraceElement[] frames = t.getStackTrace(); printStackDetails(frames); } public static void printStackDetails(StackTraceElement[] frames) { System.out.println("Frame count: " + frames.length); for (int i = 0; i < frames.length; i++) { int frameIndex = i; // i = 0 means top frame System.out.println("Frame Index: " + frameIndex); System.out.println("File Name: " + frames[i].getFileName()); System.out.println("Class Name: " + frames[i].getClassName()); System.out.println("Method Name: " + frames[i].getMethodName()); System.out.println("Line Number: " + frames[i].getLineNumber()); } } }
上面的代码生成以下结果。
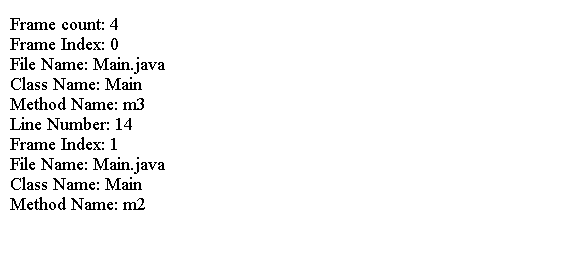
try-with-resources块
Java 7添加了一个名为try-with-resources的新结构。
使用Java 7中的新的try-with-resources构造,上面的代码可以写成
try (AnyResource aRes = create the resource...) { // Work with the resource here. // The resource will be closed automatically. }
当程序退出构造时,try-with-resources构造自动关闭资源。
资源尝试构造可以具有一个或多个catch块和/或finally块。
我们可以在try-with-resources块中指定多个资源。两个资源必须用分号分隔。
最后一个资源不能后跟分号。
以下代码显示了try-with-resources使用一个和多个资源的一些用法:
try (AnyResource aRes1 = getResource1()) { // Use aRes1 here } try (AnyResource aRes1 = getResource1(); AnyResource aRes2 = getResource2()) { // Use aRes1 and aRes2 here }
我们在try-with-resources中指定的资源是隐式最终的。
在try-with-resources中的资源必须是java.lang.AutoCloseable类型。
Java 7添加了AutoCloseable接口,它有一个close()方法。
当程序退出try-with-resources块时,将自动调用所有资源的close()方法。
在多个资源的情况下,按照指定资源的相反顺序调用close()方法。
class MyResource implements AutoCloseable { public MyResource() { System.out.println("Creating MyResource."); } @Override public void close() { System.out.println("Closing MyResource..."); } } public class Main { public static void main(String[] args) { try (MyResource mr = new MyResource(); MyResource mr2 = new MyResource()) { } } }
上面的代码生成以下结果。
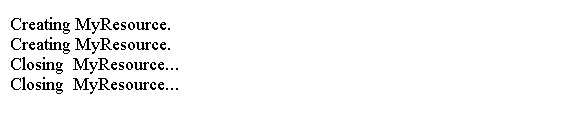
Multi-Catch块
Java 7增加了对多catch块的支持,以便在catch块中处理多种类型的异常。
我们可以在multi-catch块中指定多个异常类型。多个异常由竖线(|)分隔。
捕获三个异常:Exception1,Exception2和Exception3。
try { // May throw Exception1, Exception2, or Exception3 } catch (Exception1 | Exception2 | Exception3 e) { // Handle Exception1, Exception2, and Exception3 }
在multi-catch块中,不允许有通过子类化相关的替代异常。
例如,不允许以下multi-catch块,因为Exception1和Exception2是Throwable的子类:
try { // May throw Exception1, Exception2, or Exception3 } catch (Exception1 | Exception2 | Throwable e) { // Handle Exceptions here }